I just started learning NHibernate and I must admit that I am really enjoying *not* writing stored procedures by hand. For those who are not familiar with NHibernate you can visit the official website at NHibernate.org.
I just started learning NHibernate and I must admit that I am really enjoying *not* writing stored procedures by hand. For those who are not familiar with NHibernate you can visit the official website at NHibernate.org.
In this blog post I will briefly talk about NHibernate. Stay tunned on TheDotNetGuy for an article about NHibernate.
Let's start with creating a simple entity class.
public class Task
{
private int _id;
private string _name;
private string _description;
public virtual int ID
{
get { return _id; }
set { _id = value; }
}
public virtual string Name
{
get { return _name; }
set { _name = value; }
}
public virtual string Description
{
get { return _description; }
set { _description = value; }
}
}
And here is the mapping NHibernate file for this entity class:
<hibernate-mapping
assembly="DomainObjects"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="urn:nhibernate-mapping-2.2" >
<class name="DomainObjects.Entities.Task, DomainObjects" table="Tasks" lazy="false">
<id name="ID" column="TaskID" type="Int32">
<generator class="identity" />
</id>
<property name="Name" column="Name" type="String"/>
<property name="Description" type="String"/>
</class>
</hibernate-mapping>
For NHibernate to work we must create a "Session Factory". The session factory should be created one for each application. The reason is the complexity and the overhead behind creating the session factory. When the factory is created it loads all the information from the mapping files into the memory and that's why it is an intensive operation.
I used the Repository architecture and put the creation of session factory in the BaseRepository. Below you can see the class diagram for the Repositories.
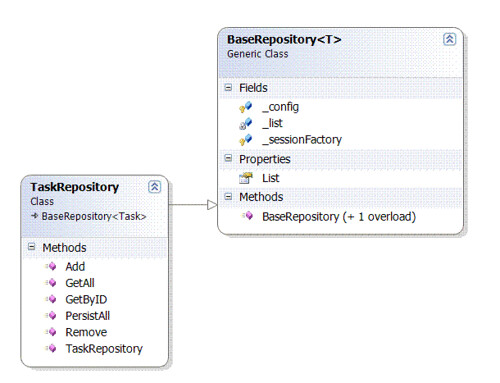
Now, let's see the methods for the TaskRepository.
public Task GetByID(int id)
{
using (ISession session = _sessionFactory.OpenSession())
{
return session.Load<Task>(id);
}
}
public IList<Task> GetAll()
{
using (ISession session = _sessionFactory.OpenSession())
{
return session.CreateCriteria(typeof(Task)).List<Task>();
}
}
Nice and simple!
This was just a very brief introduction to NHibernate. Soon, I will be writing an article which will discuss the features in detail.