Ruby provides many facilities for writing good unit tests. Although we can achieve everything in a statically typed language but Ruby just makes it a little easier. In this article we are going to discuss how to use Spec framework to perform behavior driven development on a CLR assembly using IronRuby.
Scenario:
We are going to implement a PrimeService which will return a boolean value indicating whether the passed argument was a prime number or not.
Writing Unit Tests without Spec:
Let's first check out the unit test that does not use Spec framework. We have added a new file to our project called "test_prime_without_spec.rb". Please note that the file encoding must be ANSI. We created the file in notepad and then simply added it to your project since the default text file type in notepad in ANSI.
Next we need to add references to the ruby testing framework and the CLR assembly which contains the PrimeService class. Here is the code to create the references:
Our first test will make sure that when 1 is passed it should return a true value indicating that 1 is a prime number. Here is the unit test implementation:
Couple of things to note:
1) The class name should always begin with a capital letter.
2) The test name must start with "test".
When we run the above test for the first time the test will fail because PrimeService does not exists. Let's implement the PrimeService and make this test pass.
Now, let's run the test using the command line parameters.
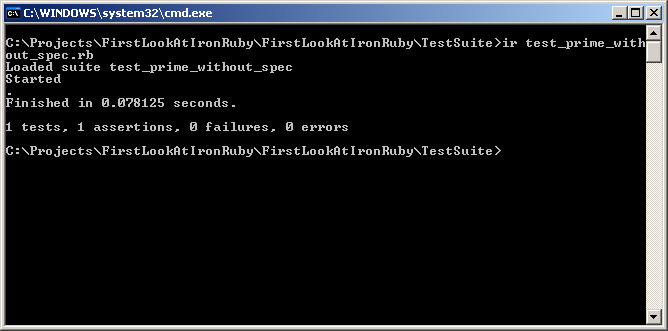
The screenshot above shows that the test passed successfully!
Although one can go and implement all the tests using the above technique but by doing so we are not acheiving anything. The above implementation is identical to what you would do in a statically typed language. To make the test more explicit let's check out Spec framework.
What is Spec?
Spec is the IronRuby implementation of the RSpec language. RSpec provides a domain specific language with which you can express executable examples of the expected behaviour of your code.
Downloading and Configuring IronRuby Version 0.6:
First, you should download the latest version of IronRuby which is version 0.6. You can download it from the following URL:
IronRuby Download Page
We have downloaded IronRuby 0.6 to the following location C:\ironruby-0.6.0. Now, let's update the path in Windows environment variables so we can execute the batch files from anywhere on the command prompt.
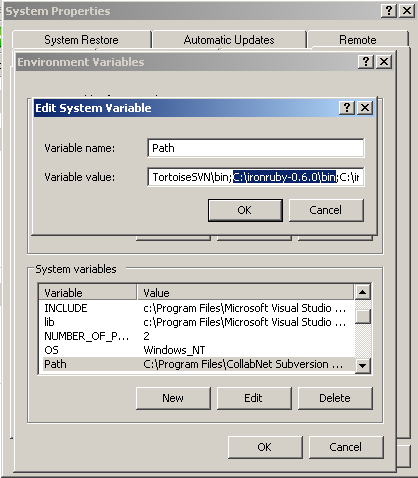
The above screenshot shows how to setup the path to the IronRuby bin folder through environment variables in Windows.
Using RubyGems to Download Spec:
The next step is to download Spec (BDD Framework) using RubyGems. Go to the bin directory of your IronRuby folder (C:\ironruby-0.6.0\bin) and run the following command.
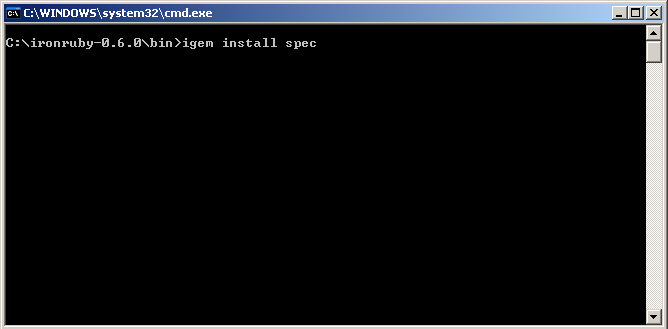
This will download the Spec framework to your machine. You can find the Spec framework batch file in the following directory.
C:\ironruby-0.6.0\lib\IronRuby\gems\1.8\bin
We will add the above path to the Windows environment variables so we can access the spec command from anywhere on the command prompt.
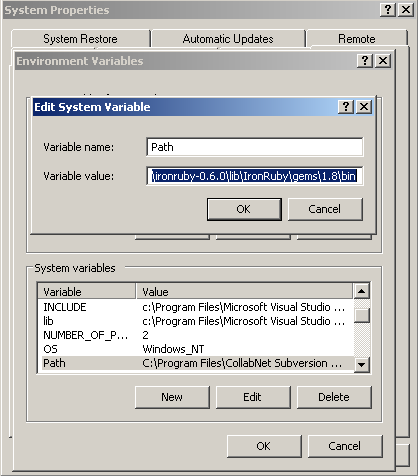
Now, we are ready to use the Spec framework to write our BDD style unit tests.
Using Spec to Write BDD Style Unit Tests:
First add the following modules which are required to use the spec framework.
We will add a reference to our CLR assembly which is contained in the bin folder of the TestSuite project.
Finally, we use the include keyword to include the BusinessObjects namespace so we don't have to write the fully qualified name of the class under test.
Here is our first test:
As, you can see we are using special domain specific language to describe our unit test. Spec frameworks provides us with these rules and makes our test more readable.
And here is the screenshot showing how to run the unit test:
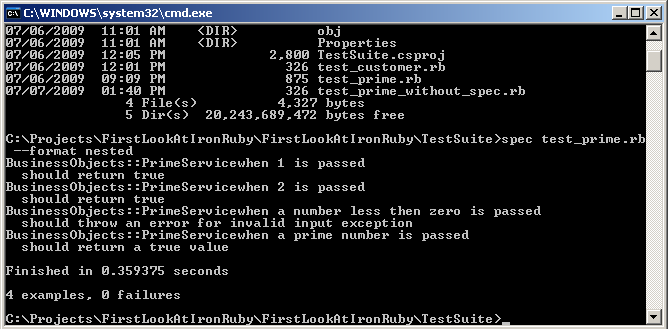
As, you can see there are 4 test also known as examples in Spec world and they all passed successfully. The format parameter is used to represent the output display for the tests. You can use nested, specdoc, html etc.
Let's take a look at the final test which ensures that if a prime number is passed to the PrimeService.IsPrime method then it should always return true.
In the above test we are passing multiple prime numbers to the IsPrime method. The result should always be true unless the list is modified to contain the non-prime numbers.
Conclusion:
In this article we learned how to unit test our CLR assembly using IronRuby. Apart from the Ruby language syntax benefits we also get benefits from Spec framework, which makes it easier to perform behaviour driven development.
UPDATE:
You can also implement the Spec BDD unit test using the following code:
The result will be displayed as follows:
C:\Projects\FirstLookAtIronRuby\FirstLookAtIronRuby\TestSuite>spec test_prime.r
--format nested
BusinessObjects::PrimeService
should return true when 1 is passed
should return true when 2 is passed
should throw an error when negative number is passed
should return true when prime number is passed
Finished in 0.359375 seconds
4 examples, 0 failures
[Download Sample]