ASP.NET MVC 3 has come a long way since its inception. One of the cool features Microsoft has added in ASP.NET MVC 3 is the idea of "Remote Validation". In this article we are going to implement username look up which uses the power of Remote Validation.
What is Remote Validation?
Remote validation allows the developer to call the controller actions using client side script. This is extremely useful when you want to perform a back end query without having to perform a full server postback.
Performing Remote Validation without ASP.NET MVC 3:
Remote validation is nothing but an Ajax call which validates the user's input. This can easily be accomplished without using ASP.NET MVC 3. The scenario for our demo is simple. We need to make sure that the username of the new user is unique. The implementation below creates a simple form which contains the TextBox control with the id "UserName".
The RegistrationViewModel is a simple ViewModel which represents the elements on the registration view. The implementation of the RegistrationViewModel is shown below:
As, you can see the RegistrationViewModel is straightforward with only a single property called "UserName". The "UserName" property is decorated with "Required" attribute which is part of DataAnnotations. You can read more about DataAnnotations attribute using the link below:
ASP.NET Validation Using DataAnnotations
Performing Validation in ASP.NET MVC Application
The heart of the code is in the view itself. The implementation below show the complete client side code to create a remote call.
The first task we performed is that we added the "blur" event to the TextBox element. The blur event will be triggered when the user will leave the TextBox. We created a params object with a dynamic "UserName" property which will hold the value from the TextBox. The $.ajax function makes a call to the ValidateUserNameManually action passing the username as the parameter. On success the response returns false which means username is already taken and a message is displayed in the DIV element as shown in the screenshot below:
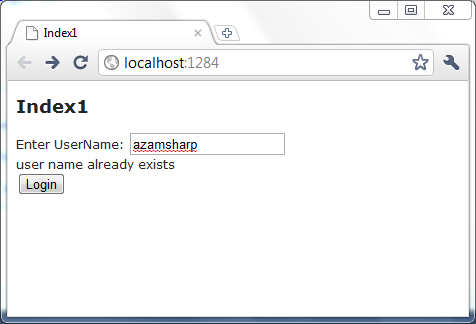
You can also check out the request in FireBug as shown below:
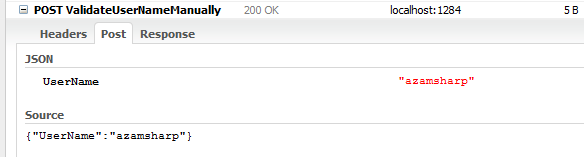
Although the remote validation was not complicated to implement but we had to write several lines of code to achieve the desired result. In the next section we are going to demonstrate how the new Remote Validation feature of ASP.NET MVC 3 can be used to ease our development.
Remote Validation in ASP.NET MVC 3:
Remote validation is achieved by using [Remote] attribute on the corresponding property of the ViewModel class. The implementation below shows the ViewModel "RegistrationViewModel" decorated with the [Remote] attribute.
The Remote attribute identifies that the "ValidateUserName" action of the "Home" controller will be invoked on the blur event. If the validation fails then the text from the "ErrorMessage" property will be displayed to the user. The "ValidateUserName" action is implemented below:
In an actual application you will use your DataAccessLayer to make sure that the username is unique. We are simply returning "false" if the username is "azamsharp".
Don't run the application just yet! You still need to refer to the client side script files which are necessary for the remote validation to work. Make sure you have reference to the following script files:
After making the changes and adding the *required* script files run the application and you will notice that whenever you lose focus from the UserName TextBox the remote validation is triggered by invoking the ValidateUserName action.
Conclusion:
In this article we learned about the new Remote Validation feature in ASP.NET MVC 3 framework. Remote validation makes it easy to call controller's action using Ajax calls. In the next article we are going to cover additional features provided by the ASP.NET MVC 3 framework.