When converting an iPhone application to an iPad application we sometimes forget that we can make use of the real estate provided by an iPad. In this article we are going to implement a dashboard app which will use the UIViewController containment feature provided by the iOS5 framework.
UIViewController Containment:
A plain UIViewController is responsible for only itself and not concerned about other controllers. Unlike UITabViewController, UISplitViewController a vanilla UIViewController cannot contain other UIViewControllers out of the box. In iOS5 framework a UIViewController has the ability to become a container for other view controllers. This is extremely beneficial for iPad applications where the larger real estate can be utilized.
Creating the Container View Controller:
Add a new UIViewController and check to use the interface xib file. Create a new “View-Based” application and do not use Storyboards. The “ViewController.h”, “ViewController.m” and “ViewController.xib” will be added by default. The ViewController will serve as our UIViewController container. Open the xib file and drag 6 UIViews on the designer. Click on the link below to see the screenshot:
UIViews on the Designer
You can use any number of UIViews you want we went ahead with six UIViews of equal sizes to utilize the complete real estate of the iPad size. Next declare all the UIViews outlets and connect them to their corresponding views using the interface builder. The declaration of IBOutlets is implemented below:
After connecting the UIViews to their IBOutlets counterparts it is time to implement the child views.
Adding Project Milestone Child View:
Add a new UITableViewController and name it “ProjectMilestoneController”. We are going to populate the UITableView with some dummy data and use custom UITableViewCell. The implementation below shows the ProjectDashboardService which fetches the dummy data.
The getProjects method simply returns some dummy data which will be consumed by our dashboard application. The cellForRowAtIndexPath is implemented below:
Depending on your data you can always use the predefined UITableViewCell templates. For this application we decided to create custom UITableViewCells which will display the data in a spreadsheet like format. The screenshot below shows the custom ProjectCell:

The UILabels separated by blank space give the illusion of the spreadsheet layout. After we have populated the UITableView with custom data we can load the ProjectMilestoneController in our container view controller.
Loading ChildViewControllers:
The iOS5 makes is very easy to load child controllers inside the container controller. The new method addChildViewController adds the child controller to the main container controller as shown in the implementation below:
The didMoveToParentViewController method sets the container controller as the parent of the newly inserted child view. The screenshot below shows the project dashboard in action:
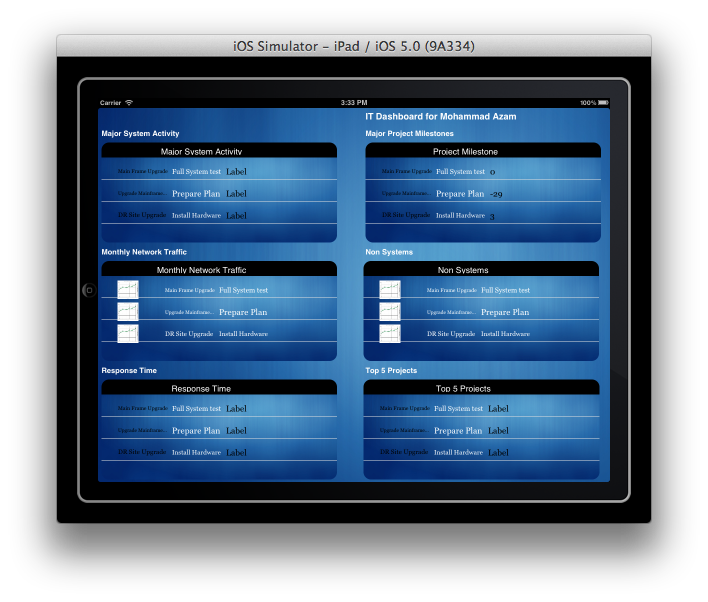
Conclusion:
In this article we learned about UIViewController containment feature which allows to load multiple UIViewControllers to a single UIViewController. In the future article we will investigate more advanced features provided by the UIViewController containment.
[Download Sample]